Export Chart
Overview
ZingChart offers the ability to export HTML5 charts into various formats (e.g., png, jpg, pdf). However, the process to accomplish the export is different for each rendering technology used. Some cases require nothing but the browser itself, while other cases require the use of an "export server," which is an online resource used to convert the chart into static formats.
Support and Technologies
Render | Formats | Method |
---|---|---|
FLASH | png , jpg , bmp , pdf |
Intrinsic Flash API |
CANVAS | png , jpg , pdf |
Intrinsic Browser API for png and jpg ; Export Server for pdf |
SVG | png , jpg , pdf |
Intrinsic Browser API for png and jpg ; Export Server for pdf |
VML | png , jpg , pdf |
Export Server |
Export Server
By default, ZingChart uses export.zingchart.com
as the export server. The export server is basically a LAMP (or similar, but with PHP installed) stack running in addition to the Batik Toolkit, a software which is able (among others) to convert SVG documents into static images or PDF files. When the image export call is issued in ZingChart (via context menu or API calls), the SVG (as string) representation of the chart is being sent to the export server and this returns the static image.
ZingChart can be configured to use other servers with the same configuration as the default Export Server. In case of sensitive data or for better speed and responsiveness, users can setup and configure their own personal Export Server and then use:
zingchart.EXPORTURL = 'http://my.server.com/path/to/index.php'; zingchart.render({ ... });
The PHP code needed to run the Batik converter can be downloaded from here.
There is another zingchart property that impacts how the library interacts with the Export Server (via one way HTTP POST or an AJAX call, see more in the API Considerations section below):
zingchart.AJAXEXPORT = true | [false];
API Considerations
The ZingChart library implements the getimagedata
API to get the base64 encoded string version of the chart's image representation.
SVG Rendering
The SVG render must use the callback function to retrieve the imagedata.
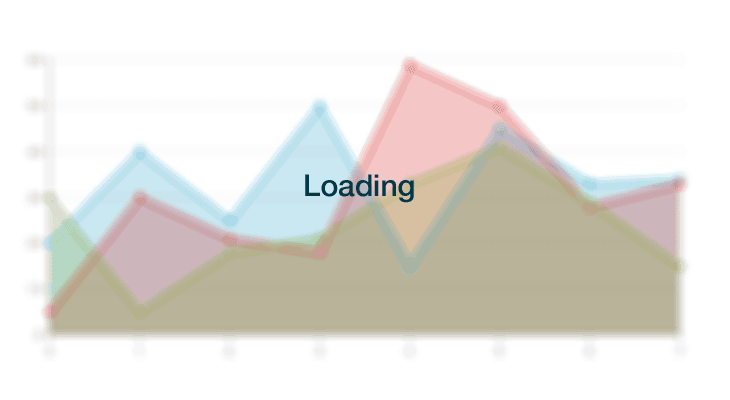
document.getElementById('test_export').addEventListener('click', function() { zingchart.exec('myChart', 'getimagedata', { filetype: 'png', callback : function(imagedata) { console.log(imagedata); document.getElementById('output_image').src = imagedata; } }); }); Copy
Canvas Rendering
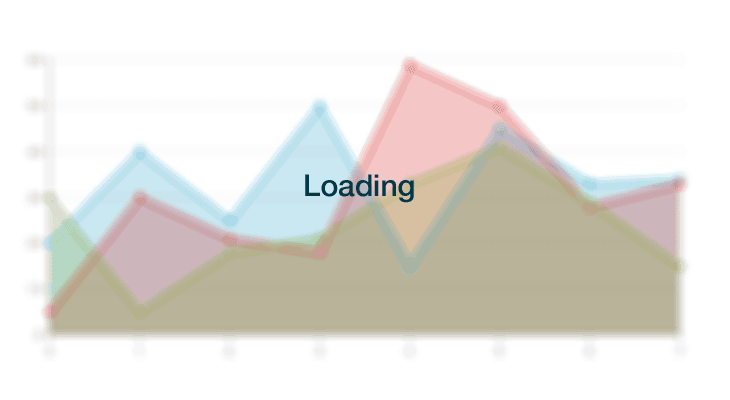
zingchart.render({ id: 'zc-demo-1', width : 400, height : 200, output : 'canvas', data: { type: 'bar', title: { text: 'Canvas render' }, series: [{ values: [3,5,8,4,2,6] }] } }); $('#zc-btn-1').click(function() { var sImageData = zingchart.exec('zc-demo-1', 'getimagedata', { format : 'png' }); $('#zc-static-1').append('<img src="' + sImageData + '">'); });
When using render mode VML (for old IE), getimagedata
will return -1 when called. This happens because even if we can get the image from the Export Server, due to cross domain security we cannot receive a response from it containing the data image; all the server can do is to "push" it to the browser. This makes the SVG rendering unsuited for projects that require image exporting.
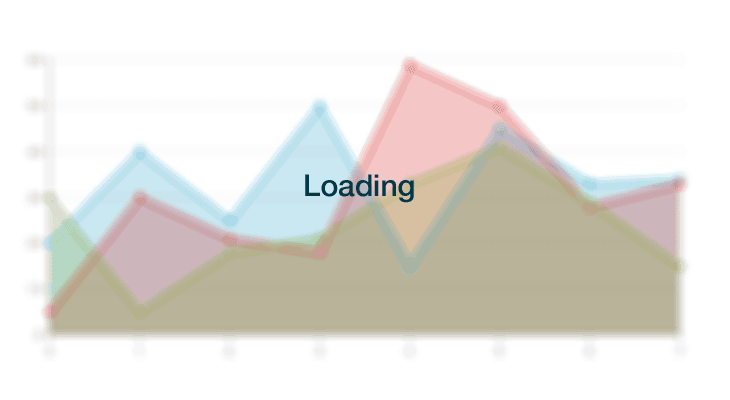
zingchart.render({ id: 'zc-demo-2', width : 400, height : 200, output : 'svg', data: { type: 'bar', title: { text: 'SVG/VML render' }, series: [{ values: [3,5,8,4,2,6] }] } }); $('#zc-btn-2').click(function() { var sImageData = zingchart.exec('zc-demo-2', 'getimagedata', { format : 'png' }); alert(sImageData); });
However, if a dedicated Export Server is installed on same domain as the chart's pages and if zingchart.AJAXEXPORT
is set to true
, the ZingChart library will perform an AJAX call and the getimagedata
API will return the base64 encoded string representing the image. The difference is that because of the AJAX request/response cycle, the result has to be processed via a callback function.
zingchart.EXPORTURL = 'http://my.server.com/'; // this will work only when the example will run under my.server.com zingchart.AJAXEXPORT = true; zingchart.exec('zc-demo-3', 'getimagedata', { format : 'png', callback : function(sImageData) { $('#zc-static-3').append('<img src="' + sImageData + '">'); } });
Summary
JavaScript chart export is a great feature of ZingChart, and can be easily implemented for any of your charts. You can export a chart using the methods outlined above, as well as our chart export API methods. Remember, you can always right-click to bring up the chart's context menu and save the chart as an image as well!